I could not find a sample in the documentation to initialize a custom BarHistory. The documentation does not have any sample code near the BarHistory constructor. Can you please share how we can create a custom BarHistory object and plot it?
This is what I tried (non working):
Also for the PlotBarHistoryStyle - how do I plot up bars and down bars with different colors? The API shows only one option for color.
What are valid values for "styleName" in PlotBarHistoryStyle (probably a list of valid values should be added to the documentation).
This is what I tried (non working):
CODE:
public override void Initialize(BarHistory bars) { BarHistory extBars = GetHistory(bars, "TSLA"); var scaleFactor = 0.36; BarHistory extBarsScaled = new BarHistory(extBars); extBarsScaled.Open = extBars.Open * scaleFactor; extBarsScaled.High = extBars.High * scaleFactor; extBarsScaled.Low = extBars.Low * scaleFactor; extBarsScaled.Close = extBars.Close * scaleFactor; PlotBarHistoryStyle(extBarsScaled, "price", "bars", Color.Black, false); }
Also for the PlotBarHistoryStyle - how do I plot up bars and down bars with different colors? The API shows only one option for color.
What are valid values for "styleName" in PlotBarHistoryStyle (probably a list of valid values should be added to the documentation).
Rename
Here's how it should be -- using the (documented) .Add method:
CODE:
//create indicators and other objects here, this is executed prior to the main trading loop public override void Initialize(BarHistory bars) { BarHistory extBars = GetHistory(bars, "TSLA"); var scaleFactor = 0.36; BarHistory extBarsScaled = new BarHistory(extBars); for (int i = 0; i < extBars.Count; i++) { extBarsScaled.Add(extBars.DateTimes[i], extBars.Open[i] * scaleFactor, extBars.High[i] * scaleFactor, extBars.Low[i] * scaleFactor, extBars.Close[i] * scaleFactor, 0); } PlotBarHistoryStyle(extBarsScaled, "price", "bars", Color.Black, false); }
Thank you Eugene!
That works!
Any ideas on these:
> Also for the PlotBarHistoryStyle - how do I plot up bars and down bars with different colors? The API shows only one option for color.
> What are valid values for "styleName" in PlotBarHistoryStyle (probably a list of valid values should be added to the documentation).
That works!
Any ideas on these:
> Also for the PlotBarHistoryStyle - how do I plot up bars and down bars with different colors? The API shows only one option for color.
> What are valid values for "styleName" in PlotBarHistoryStyle (probably a list of valid values should be added to the documentation).
You can use the ChartStyleFactory.ChartStyles to get a list of installed chart styles, each such ChartStyle instance has a Name property.
We’ll have to add an override to allow you to plot up and down bar colors.
We’ll have to add an override to allow you to plot up and down bar colors.
I was exploring on Visual Studio to try to understand why
So the extBarsScaled.Open TimeSeries is "get" but not "set". Perhaps it would be nice to make TimeSeries.Open, .Close, .High, and .Low settable too so the solution in the original post works? This would be more efficient because the .Add operation has to add more storage individually for each .Add executed.
The fancy solution would be to wrap the solution in the original post into a BarHistory constructor and allow that constructor (via "protected" set) to perform the TimeSeries set operation. But that's more development work. :(
CODE:doesn't work because I thought it would.
extBarsScaled.Open = extBars.Open * scaleFactor;
So the extBarsScaled.Open TimeSeries is "get" but not "set". Perhaps it would be nice to make TimeSeries.Open, .Close, .High, and .Low settable too so the solution in the original post works? This would be more efficient because the .Add operation has to add more storage individually for each .Add executed.
The fancy solution would be to wrap the solution in the original post into a BarHistory constructor and allow that constructor (via "protected" set) to perform the TimeSeries set operation. But that's more development work. :(
I doubt it's a good idea to make these properties settable.
QUOTE:I tend to agree (And you knew I would.) because that can create "side effects" when foreign code monkeys around with class-library objects (WL objects). The problem is these introduced side effects aren't realized until 1000s of execution lines later, which means you need to lint the source code to find out what foreign code is corrupting things. (And I doubt the WL user with a 166-thousand line strategy has a C# lint utility.)
I doubt it's a good idea to make these properties settable.
Adding a new BarHistory constructor is the safer solution, but that may have to wait until WL 7.1. :(
BTW, I experimented with setting float.NaN to price bars and that actually helped me with the following solution for plotting custom bar history with two colors:
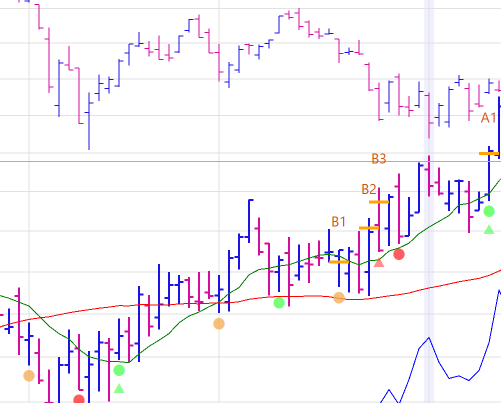
Thanks for adding "stacking" to bar annotations in Build 6. I can already see it the chart.
My next question - how do I add annotations to the secondary bar history?
I think secondary, or tertiary bar histories should have all the charting capability as the primary ones. My primary bar is already too crowded and will like to move some annotations (which belongs to the secondary one) to the secondary one.
CODE:
BarHistory SPXScaledUp = new BarHistory(SPX); BarHistory SPXScaledDown = new BarHistory(SPX); for (int i = 0; i < SPX.Count; i++) { if (SPX.Close[i] >= SPX.Open[i]) { SPXScaledUp.Add(SPX.DateTimes[i], SPX.Open[i] * factor, SPX.High[i] * factor, SPX.Low[i] * factor, SPX.Close[i] * factor, SPX.Volume[i]); SPXScaledDown.Add(SPX.DateTimes[i], float.NaN, float.NaN, float.NaN, float.NaN, float.NaN); } else { SPXScaledUp.Add(SPX.DateTimes[i], float.NaN, float.NaN, float.NaN, float.NaN, float.NaN); SPXScaledDown.Add(SPX.DateTimes[i], SPX.Open[i] * factor, SPX.High[i] * factor, SPX.Low[i] * factor, SPX.Close[i] * factor, SPX.Volume[i]); } } PlotBarHistoryStyle(SPXScaledUp, "Price", "bars", this.settings.upColor, false); PlotBarHistoryStyle(SPXScaledDown, "Price", "bars", this.settings.downColor, false);
Thanks for adding "stacking" to bar annotations in Build 6. I can already see it the chart.
My next question - how do I add annotations to the secondary bar history?
I think secondary, or tertiary bar histories should have all the charting capability as the primary ones. My primary bar is already too crowded and will like to move some annotations (which belongs to the secondary one) to the secondary one.
I "think" the BarHistory constructor already sets Open, Close, etc. to Double.NaN by default, so you don't need to do that yourself. Just use .Add to set what's not a Double.NaN.
Also, Float.NaN is for single precision, and WL is using double precision. So you want to set undefined data to Double.NaN instead.
Now "some" compilers have switch settings that can change Float from single to double precision with the right switch setting. You'll need to research the C# switch settings for more information about that.
Also, Float.NaN is for single precision, and WL is using double precision. So you want to set undefined data to Double.NaN instead.
Now "some" compilers have switch settings that can change Float from single to double precision with the right switch setting. You'll need to research the C# switch settings for more information about that.
Your Response
Post
Edit Post
Login is required