I have a script which shows charts for two symbols as follows:
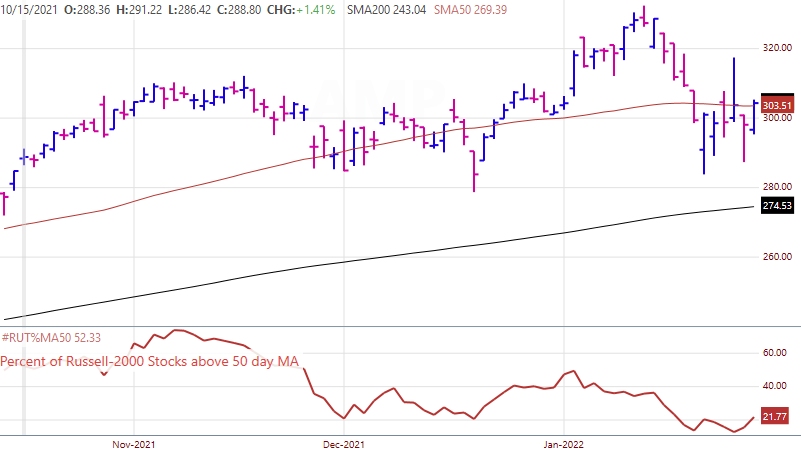
This is the code:
I can change the symbol for the primary chart by clicking on any symbol in the sidebar or typing a symbol in the symbol box. I am looking for ways to change the symbol for the secondary chart without modifying the code or having multiple copies of the same script. Any ideas?
This is the code:
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Indicators; using System.Drawing; using System.Collections.Generic; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { //create indicators and other objects here, this is executed prior to the main trading loop public override void Initialize(BarHistory bars) { Color color50d = Color.FromArgb(255, 230, 70, 60); PlotTimeSeriesLine(SMA.Series(bars.Close, 50), "SMA50", "Price", color50d, 1); PlotTimeSeriesLine(SMA.Series(bars.Close, 200), "SMA200", "Price", Color.Black, 1); // Stocks in Russell 2000 above 200 MA BarHistory bars2 = GetHistory(bars, "#RUT%MA50"); PlotBarHistoryStyle(bars2, "paneBottom", "line", color50d); DrawHeaderText("Percent of Russell-2000 Stocks above 50 day MA",color50d, 14, "paneBottom"); } //execute the strategy rules here, this is executed once for each bar in the backtest history public override void Execute(BarHistory bars, int idx) { if (!HasOpenPosition(bars, PositionType.Long)) { //code your buy conditions here } else { //code your sell conditions here } } //declare private variables below } }
I can change the symbol for the primary chart by clicking on any symbol in the sidebar or typing a symbol in the symbol box. I am looking for ways to change the symbol for the secondary chart without modifying the code or having multiple copies of the same script. Any ideas?
Rename
1. Your strategy could pop up an dialog box and wait for your input like this:
https://www.wealth-lab.com/Discussion/InputBox-6392
2. There's more than one way, of course. For example, your code could parse an external file for input etc.
https://www.wealth-lab.com/Discussion/InputBox-6392
2. There's more than one way, of course. For example, your code could parse an external file for input etc.
I think the most straightforward way is to create a Dictionary and hard-code the symbols in the script. If the list is long, then you could load the dictionary from a file.
Note that in the code, the symbols "on the left" are the "keys" to the Dictionary. They must be unique. On the right is the secondary symbol you assign to them - these can be duplicates.
Note that in the code, the symbols "on the left" are the "keys" to the Dictionary. They must be unique. On the right is the secondary symbol you assign to them - these can be duplicates.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Indicators; using System.Drawing; using System.Collections.Generic; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { public override void Initialize(BarHistory bars) { _lookup = new Dictionary<string, string>(); _lookup["AAPL"] = "#RUT%MA50"; _lookup["TTD"] = "#RUT%MA50"; _lookup["ZS"] = "QQQ"; _lookup["IBM"] = "SPY"; _lookup["SIRI"] = "QQQ"; _lookup["CSCO"] = "SPY"; _secondsym = _lookup[bars.Symbol]; Color color50d = Color.FromArgb(255, 230, 70, 60); PlotTimeSeriesLine(SMA.Series(bars.Close, 50), "SMA50", "Price", color50d, 1); PlotTimeSeriesLine(SMA.Series(bars.Close, 200), "SMA200", "Price", Color.Black, 1); // Stocks in Russell 2000 above 200 MA BarHistory bars2 = GetHistory(bars, _secondsym); PlotBarHistoryStyle(bars2, "paneBottom", "line", color50d); DrawHeaderText("Percent of Russell-2000 Stocks above 50 day MA", color50d, 14, "paneBottom"); } //execute the strategy rules here, this is executed once for each bar in the backtest history public override void Execute(BarHistory bars, int idx) { if (!HasOpenPosition(bars, PositionType.Long)) { //code your buy conditions here } else { //code your sell conditions here } } //declare private variables below Dictionary<string, string> _lookup; string _secondsym; } }
So we've got three ways and counting 😃
Thank you Eugene and Cone!!!
Both are good ideas!
On the UI popup, I have to think more on a way to streamline it with my workflow.
The symbol mapping will work if I have only one-to-many relations from primary symbol to secondary symbol.
I think this feature will make it work for all combinations (many-to-many) - https://www.wealth-lab.com/Discussion/Add-Symbol-Mappings-for-Historical-Providers-7000
I have added comment in that thread and voted it!
Both are good ideas!
On the UI popup, I have to think more on a way to streamline it with my workflow.
The symbol mapping will work if I have only one-to-many relations from primary symbol to secondary symbol.
I think this feature will make it work for all combinations (many-to-many) - https://www.wealth-lab.com/Discussion/Add-Symbol-Mappings-for-Historical-Providers-7000
I have added comment in that thread and voted it!
Your Response
Post
Edit Post
Login is required